(Perl:
Lesson 6)
{ Parsing /etc/passwd
with split }
Section 0. Background
Information |
- What is Perl
- Perl is a high-level, general-purpose,
interpreted, dynamic programming language. Perl was originally developed
by Larry Wall in 1987 as a general-purpose Unix scripting language to
make report processing easier. Since then, it has undergone many
changes and revisions and become widely popular amongst programmers.
Larry Wall continues to oversee development of the core language, and
its upcoming version, Perl 6. Perl borrows features from other
programming languages including C, shell scripting (sh), AWK, and sed.
The language provides powerful text processing facilities without the
arbitrary data length limits of many contemporary Unix tools,
facilitating easy manipulation of text files. Perl gained widespread
popularity in the late 1990s as a CGI scripting language, in part due to
its parsing abilities.
- Getting Perl
- For the purposes of these perl lesson, I
will be using a perl package that comes standard on Backtrack, Ubuntu
and most flavors of Linux and Unix.
- However, if you are using Windows, instead
of a Linux, Unix or MAC operating system, you still have options.
- Pre-Requisite
-
Lab
Notes
- In this lab we will do the following:
- We will download a program that
contains a subroutine.
- The program will read the /etc/passwd
file into an @ARRAY.
- Then the program will enumerate through
each line of the @ARRAY (a.k.a., /etc/passwd) and use split to
extract element of the line into the following variables.
-
my($username,$passwd,$uid,$guid,$geco,$homedir,$shell) =
split(/:/,$line);
- Legal Disclaimer
- As a condition of your use of this Web
site, you warrant to computersecuritystudent.com that you will not use
this Web site for any purpose that is unlawful or
that is prohibited by these terms, conditions, and notices.
- In accordance with UCC § 2-316, this
product is provided with "no warranties, either express or implied." The
information contained is provided "as-is", with "no guarantee of
merchantability."
- In addition, this is a teaching website
that does not condone malicious behavior of
any kind.
- Your are on notice, that continuing
and/or using this lab outside your "own" test environment
is considered malicious and is against the law.
- © 2013 No content replication of any
kind is allowed without express written permission.
Section 1.
Login to BackTrack |
- Start Up VMWare Player
- Instructions:
- Click the Start Button
- Type Vmplayer in the search box
- Click on Vmplayer
-
- Open a Virtual Machine
- Instructions:
- Click on Open a Virtual Machine
-
- Open the BackTrack5R1 VM
- Instructions:
- Navigate to where the BackTrack5R1 VM
is located
- Click on on the BackTrack5R1 VM
- Click on the Open Button
-
- Edit the BackTrack5R1 VM
- Instructions:
- Select BackTrack5R1 VM
- Click Edit virtual machine settings
-
- Edit Virtual Machine Settings
- Instructions:
- Click on Network Adapter
- Click on the Bridged Radio button
- Click on the OK Button
- Play the BackTrack5R1 VM
- Instructions:
- Click on the BackTrack5R1 VM
- Click on Play virtual machine
-
- Login to BackTrack
- Instructions:
- Login: root
- Password: toor or <whatever you changed
it to>.
-
- Bring up the GNOME
- Instructions:
- Type startx
-
Section 2.
Bring up a
console terminal |
- Start up a terminal window
- Instructions:
- Click on the Terminal Window
- Obtain the IP Address
- Instructions:
- ifconfig -a
- Note(FYI):
- My IP address 192.168.1.111.
- In your case, it will probably be
different.
-
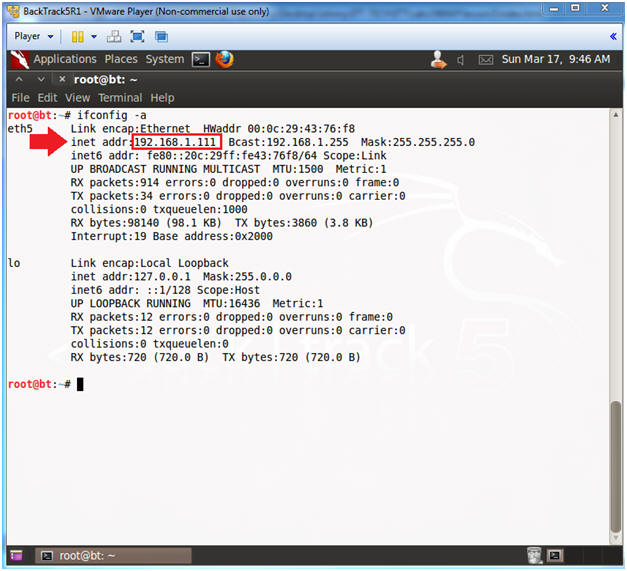
Section 3.
Download lesson6a.pl |
- Become the student user and make a directory
- Instructions:
- su - student
- mkdir -p perl_lessons
- cd perl_lessons
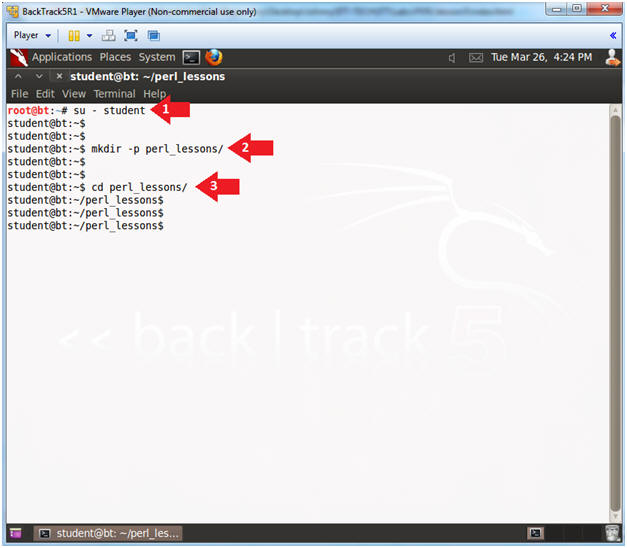
- Download lesson6a.pl
- Instructions:
- wget http://www.computersecuritystudent.com/UNIX/PERL/lesson6/lesson6a.pl
- chmod 700 lesson6a.pl
- perl -c lesson6a.pl
- Run lesson6a.pl
- Instructions:
- ./lesson6a.pl
- Note(FYI):
- Before continuing to the proof of lab
section.
- Read each line of the code and examine
how each subroutine executes.
- Then read the following "Analyze The
Code" section.
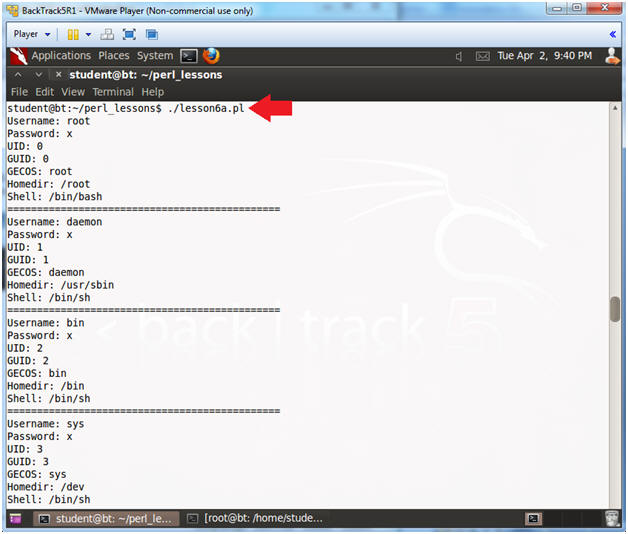
Section 6.
Analyze The Code |
- SheBang Directive
- Instructions:
- vi lesson6a.pl
- :set nu
- Press the <Enter> key
- Note(FYI):
- Line 1: #!/usr/bin/perl
- #! - is called the SheBang Directive.
SheBang is an interpreter directive that tells Linux to load the
following program.
- /usr/bin/perl - is the Perl
Interpreter. SheBang tells the program loader to run the Perl
Interpreter.
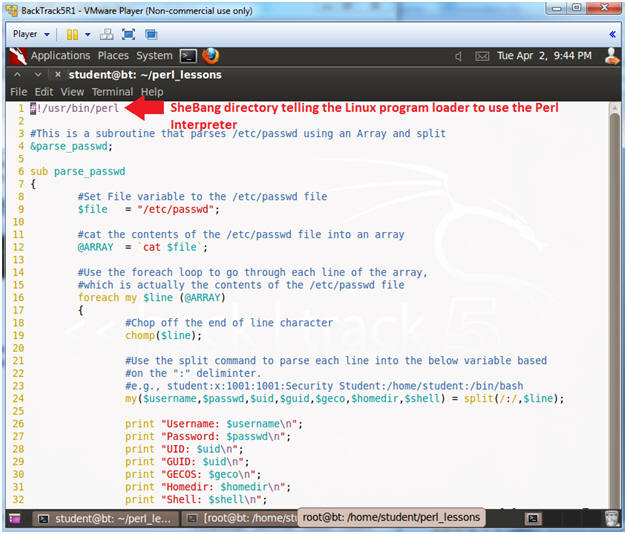
- Explaining Lines 6 through 13
- Instructions:
- Arrow down to line 6
- Note(FYI):
- Line 4: &parse_passwd;
- Call subroutine &parse_passwd to
execute.
- Line 6: sub parse_passwd
- parse_passwd is the name of the
subroutine.
- Line 7: {
- Start of the parse_passwd subroutine
- Lines 9: $file = "/etc/passwd";
- Set variable $file to the actual /etc/passwd
file.
- Line 12: @ARRAY = `cat $file`;
- Cat the content of the variable $file
to the @ARRAY data structure. i.e., The array will contain the
contents of /etc/passwd.
- Explaining Lines 14 through 20
- Instructions:
- Arrow down to line 16
- Note(FYI):
- Line 16: foreach my $line (@ARRAY)
- This a foreach loop, that
sequentially moves line by line through the array.
- Line 17: {
- Start of the foreach loop
- Line 39: }
- Line 19: chomp($line);
- chomp is a perl function use to chop
off any newline characters at the end of the line.
- The variable $line is assign to each
line of the @ARRAY as it sequentially enumerates.
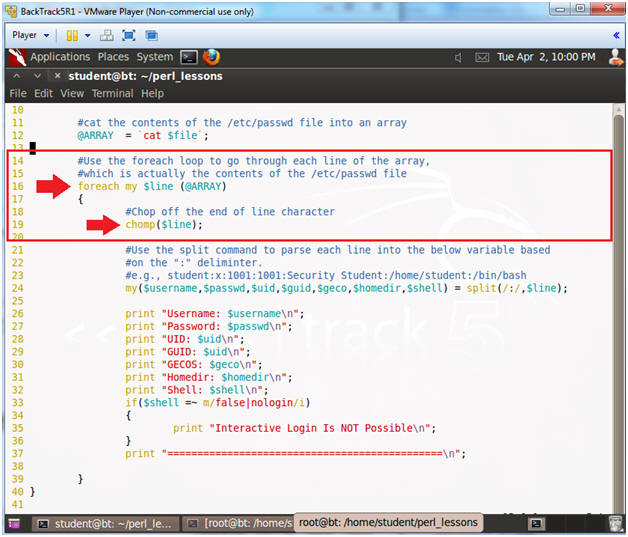
- Explaining Lines 21 through 24
- Instructions:
- Arrow down to line 24
- Note(FYI):
- Line 24:
my($username,$passwd,$uid,$guid,$geco,$homedir,$shell) =
split(/:/,$line);
-
Split
is a built-in perl function that is used to break up strings into
substrings based on a delimiter.
- A delimiter is a character(s) that
separates elements of a string. The below example is the first
line in the /etc/passwd file. Notice that ":"
is the delimiter that separates each element of the /etc/passwd
file.
- e.g., root:x:0:0:root:/root:/bin/bash
- Split up variable $line by delimiter
":" into variables $username,$passwd,$uid,$guid,$geco,$homedir,$shell.
- Notice there are six ":"
delimiters which separates seven data elements.
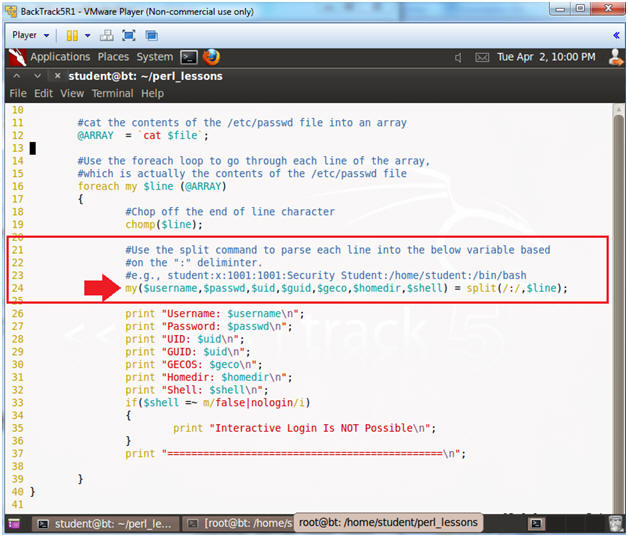
- Explaining Lines 26 through 32
- Instructions:
- Arrow down to line 26
- Note(FYI):
- Lines 26 - 32: Multiple Print Statement
- Print the contents of each variable.
- Explaining Lines 33 through 40
- Instructions:
- Arrow down to line 33
- Note(FYI):
- Lines 33 - 36: if($shell =~ m/false|nologin/i)
-
IF
the variable $shell contains the word false or nologin,
THEN
print "Interactive Login Is NOT Possible".
- Line 39: }
- Line 40: }
- End of the subroutine parse_passwd
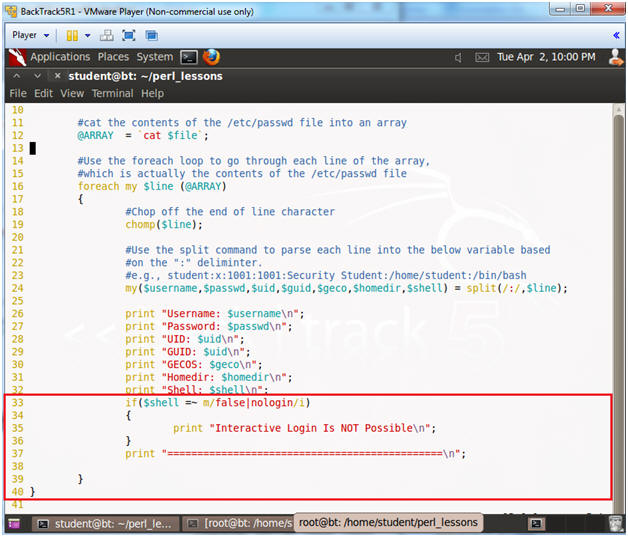
- Save and Quit
- Instructions:
- Press the <Esc> key
- :q!
- Press the <Enter> key
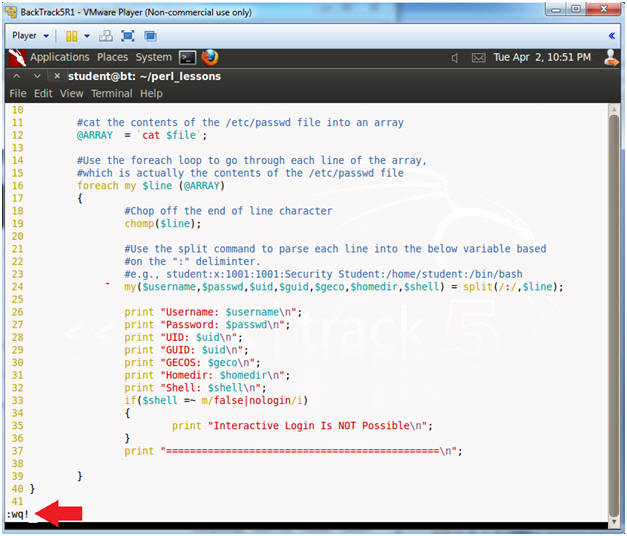
- Project
- Instructions:
- cp lesson6a.pl lesson6b.pl
- Make your edits in lesson6b.pl
- Basically, you will modify the existing
code. You will leave program pretty much intact including the
subroutine, foreach loop, split, if statement, and prints.
- The program should prompt the user to
search for a username.
- e.g., print "Username Search: ";
- The program should assign variable
$search to standard input
- e.g., chomp(my $search = <stdin>);
- The program will contain the same subroutine
called parse_passwd, but it will take in the variable $search as a
parameter.
- e.g., &parse_passwd($search);
- After the line that contains split, the
program should immediately contains an IF statement that allows you
to see if the $username variable contains the $search variable.
- e.g., if($username =~ m/$search/i)
- Inside the IF statement, place the
following print statements. The below print statements should
go between the opening starting
{
brace after the IF statement and the closing
} brace.
- print ">>>>>>> Match Found
<<<<<<<<<\n";
- print "Username: $username\n";
- print "Password: $passwd\n";
- print "UID: $uid\n";
- print "GUID: $uid\n";
- print "GECOS: $geco\n";
- print "Homedir: $homedir\n";
- print "Shell: $shell\n";
- Proof of Lab
- Instructions
- chmod 700 lesson6b.pl
- perl -c lesson6b.pl
- ./lesson6b.pl
- date
- echo "Your Name"
- Put in your actual name in place of
"Your Name"
- e.g., echo "John Gray"
- Do a PrtScn, Paste into a word
document, and upload to Moodle.
-
Proof Of Lab
Instructions:
- Press the PrtScn key
- Paste into a word document
- Upload to Moodle
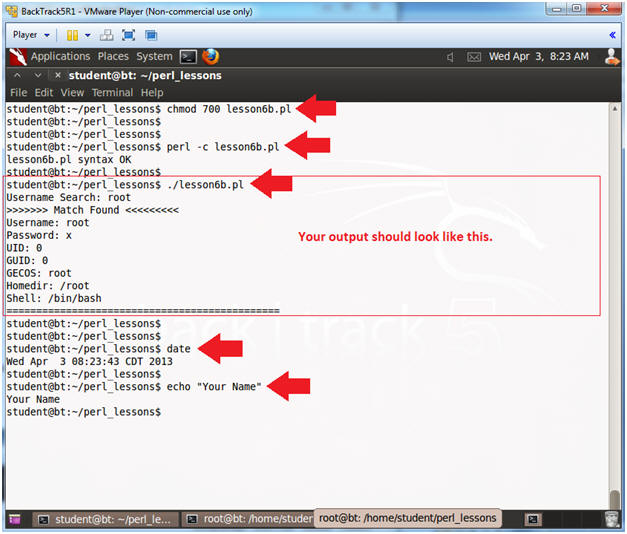
|

 
|